Lava Lamp Shader
attempting to replicate a lava lamp through ray marching in unity
Overview:
ray marching is a rendering technique used to visualize things like mathematical functions inside a volume by iteratively sampling along a ray to find the nearest surface intersection. this shader utilizes ray marching to render spheres dynamically, creating a lava lamp effect. the following sections detail the key components of the raymarching implementation.
Components:
Ray Initialization:
-
The ray origin calculation computes the starting point of the ray in object space using the camera's world position as its input. this function converts the camera's world position into object space using unity_WorldToObject. This outputs the position of the object space from which the ray begins through the variable rayOrigin.
-
The ray direction calculation determines the direction of the ray based on the pixel's position. this function takes the position of the current pixel referred to as the hitposition and the rayOrigin outputted from the ray origin calculation. the calculation normalizes the vector of the rayOrigin to the hitposition and outputs the rayDirection variable which is the direction vector of the ray.​
Distance Function:
-
The signed distance function provides the signed distance from a point in space to the nearest sphere surface, it does this by calculating distances to each sphere and finding the closest surface to it.
-
The inputs it takes are the animated positions of the spheres, the radii of the spheres, and the static sphere positions. with these, it computes the distance to each sphere using distanceToSphere and uses smoothMin to blend distance between spheres, creating smoother transitions.. the output of this is the distance from the current position to the closest surface of any sphere.​
Ray Marching Algorithm:
-
The ray marching algorithm iteratively marches along the ray to find the surface intersection or determine fi the ray exits the scene. the main parameters it takes are the starting point of the ray and the direction of the ray.
-
Firstly, the distance from the origin and the distance from the scene are initialized and are then iterated up to the maximum steps, where finally if the maximum distance is exceeded or it is close enough to the surface the loop is exited.
-
Additionally, this outputs the total distance travelled by the ray before hitting the surface or exiting ​
Fragment Shader:
-
The fragment shader determines the final colour based on the ray marching results.
-
If the compute distance is within the maximum distance, the hit position is computed and the colour is interpolated based on the hit position's 'y' coordinate using a colour gradient and if the computed distance exceeds the maximum distance, discard the fragment.
​​
​
Constants and parameters:
-
'MAX_STEPS': maximum number of steps for ray marching to avoid infinite loops and control the rendering quality
-
'MAX_DIST': maximum distance the ray can travel before it is considered to have missed the surface
-
'SURF_DIST': threshold distance at which the ray considers itself to have hit the surface, defining the precision of the surface detection
​​
​
Performance considerations:
-
Increasing 'MAX_STEPS' improves accuracy but affects performance. Optimize based on scene complexity.
-
Adjust 'MAX_DIST' and 'SURF_DIST' to balance between visual quality and computational efficiency(use case by use case basis).
​​
​
Possible future improvements:
1. Instead of defining each sphere's properties individually, it might be a good idea to utilize arrays for scalability and ease of managements was I to add more spheres.
2. adaptive step sizing and a method to visualize the ray marching would help in things like diagnosing certain issues as well as generally making the code more adaptable.
3. the animation calculation logic could be moved into a separate function to for ease of adjustability.
4. make sure all precision qualifiers for floats and vectors are correct for the scenario.
5. find an alternative method rather than using multiple.
6. adding a function to make the lava lamp blobs work with a more traditional lava lamp shape through things such as changing the volume shape or clamping the blob attributes so that they will always be narrower at the top to prevent clipping.
​
​
attributes exposed in the inspector:
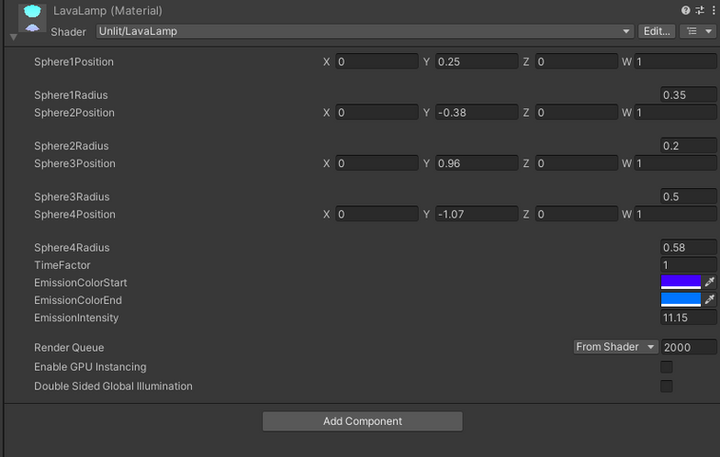
Result of initial ray marching test:
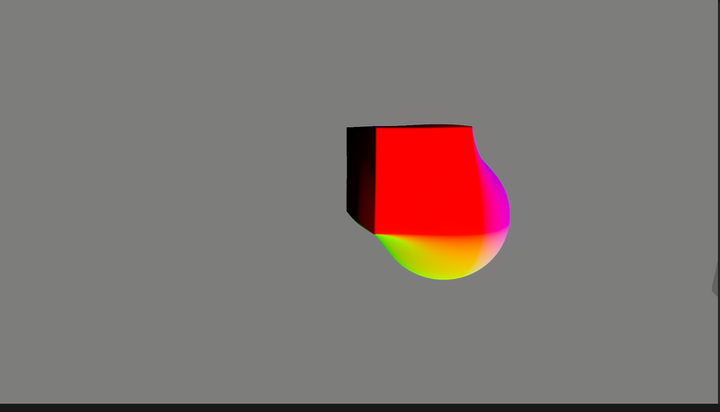
Result of the finalized lava lamp:
